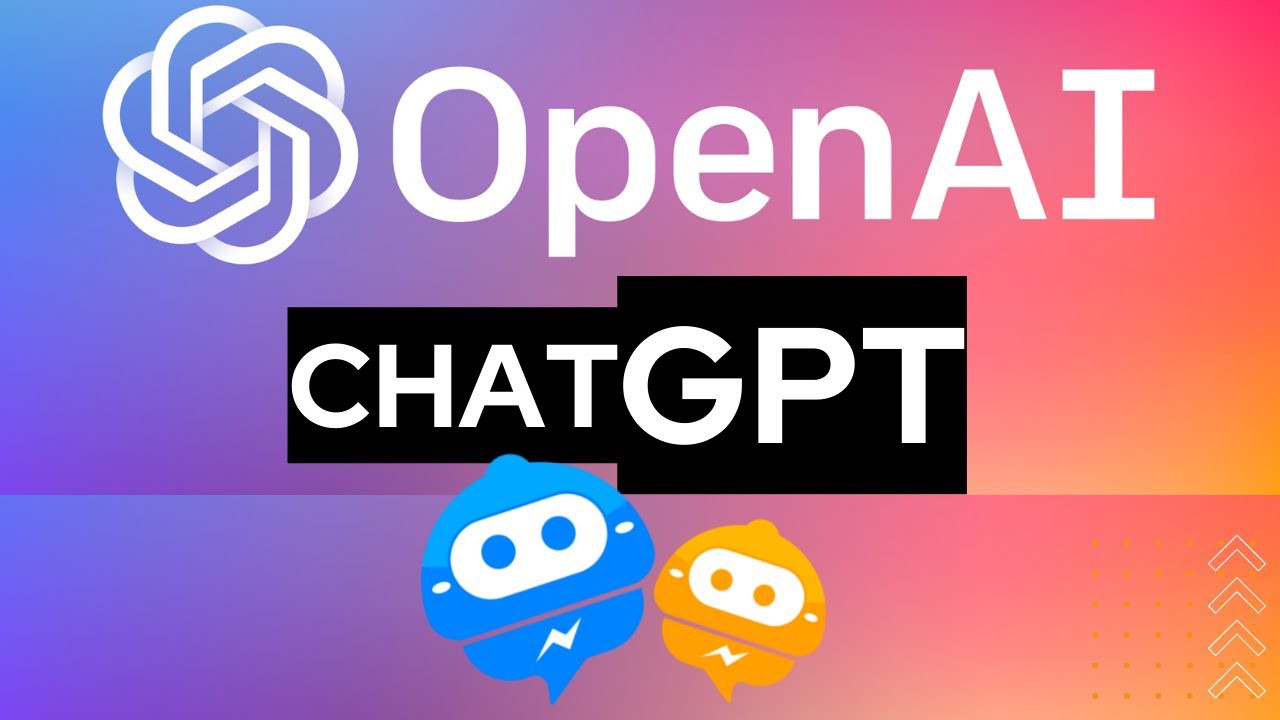
To connect to ChatGPT from Python, you can use OpenAI’s openai
library, which allows you to easily interact with the OpenAI API. Here is an example of how to use the openai
library to connect to ChatGPT and generate a response to a prompt using Python:
import openai
# Set the API key
openai.api_key = "YOUR_API_KEY"
# Define the prompt
prompt = "Hello, how can I help you today?"
# Use the `openai.Completion.create()` method to generate a response from ChatGPT
response = openai.Completion.create(
engine="text-davinci-002",
prompt=prompt,
max_tokens=1024,
n=1,
stop=None,
temperature=0.5,
)
# Print the response
print(response["choices"][0]["text"])
It’s important to note that you’ll need to replace "YOUR_API_KEY"
with your own API key, which you can obtain by creating an account on the OpenAI website.
You also need to have the package openai
installed on your system, you can install it via pip:
pip install openai
Also, you can use Hugging Face’s transformers
library (here), which allows you to easily interact with various pre-trained language models including GPT-2, GPT-3, etc. Here is an example of how to use the transformers
library to generate a response from GPT-3
fromtransformers import GPT3Tokenizer, GPT3ForCausalLM
# Instantiate the tokenizer
tokenizer = GPT3Tokenizer.from_pretrained("gpt3")
# Instantiate the model
model = GPT3ForCausalLM.from_pretrained("gpt3")
# Define the prompt
prompt = "Hello, how can I help you today?"
# Tokenize the prompt
encoded_prompt = tokenizer.encode(prompt, return_tensors='pt')
# Generate a response
response = model.generate(input_ids=encoded_prompt, max_length=1024)
# Decode the response
decoded_response = tokenizer.decode(response[0], skip_special_tokens=True)
# Print the response
print(decoded_response)
It’s important to note that you’ll need to have the API key for GPT-3 and also to have an internet connection to run the above code snippet. Also, you’ll need to have the package transformers
installed on your system, you can install it via pip:
pip install transformers
Both libraries, openai
and transformers
are widely used to interact with pre-trained language models, you can choose the one that fits your needs better.
That is all for today!
Hope you find this post and the associated information useful.
I would encourage you to post any questions or share your thoughts on this topic in the comments section below.
Leave a Reply Cancel reply